What's new in JavaScript?

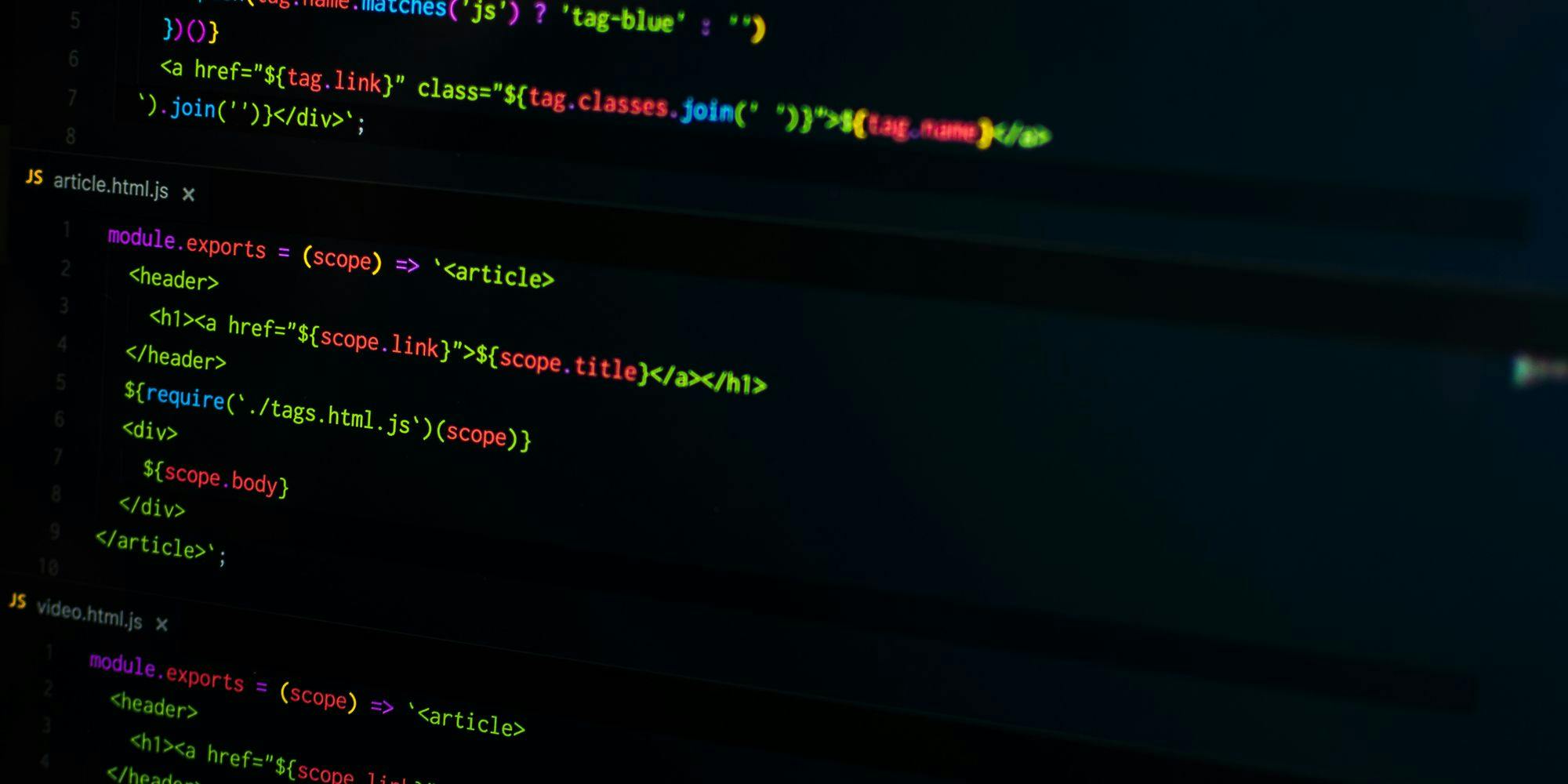

ECMAScript 2015 (also known as ES6) and later introduced some of the following features:
1. let and const:
These two new keywords were introduced for variable declaration
let variableOne = 'I can be updated.';
const variableTwo = 'I cannot be updated.';
2. Template literals:
Template literals provide an easy way to interpolate variables and expressions within a string
let name = 'John';
let age = 25;
let message = `Hello, my name is ${name} and I am ${age} years old.`;
3. Arrow functions:
Arrow functions introduce a shorter syntax for defining functions
// Traditional function
function add(x, y) {
return x + y;
}
// Arrow function
const add = (x, y) => x + y;
4. Promises:
Promises were introduced for better handling of asynchronous operations
let myPromise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Promise resolved');
}, 5000);
});
myPromise.then((value) => {
console.log(value);
});
5. Async/await:
This was introduced in ES2017 to simplify working with promises
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
}
fetchData();
6. Object de-structuring:
This allows you to extract properties from objects in a more concise way
let person = {
name: 'Alice',
age: 30
};
const {name, age} = person;
console.log(`${name} is ${age} years old.`);
7. Array de-structuring:
This allows you to extract elements from an array in a more concise way
let numbers = [1, 2, 3];
let [first, , third] = numbers;
console.log(`${first} and ${third} are the first and third elements.`);
8. Spread syntax:
The spread syntax allows you to expand an iterate-able (like an array or an object) into individual elements
let arrayOne = [1, 2, 3];
let arrayTwo = [4, 5, 6];
let combinedArray = [...arrayOne, ...arrayTwo];
console.log(combinedArray); // [1, 2, 3, 4, 5, 6]
9. Default parameters:
Allows you to set default values for function parameters
function greet(name = 'World') {
console.log(`Hello, ${name}!`);
}
greet(); // Outputs: Hello, World!
greet('John'); // Outputs: Hello, John!
10. Rest parameters:
Captures a variable number of function arguments into an array
function sum(...numbers) {
return numbers.reduce((total, n) => total + n, 0);
}
console.log(sum(1, 2, 3, 4)); // Outputs: 10
11. Classes:
Introduces a more object-oriented way to define classes and inheritance
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
const dog = new Dog('Rover');
dog.speak(); // Outputs: Rover barks.
12. Modules:
Native support for importing and exporting functionalities in separate files
// file: utils.js
export function square(x) {
return x * x;
}
// file: main.js
import { square } from './utils';
console.log(square(3)); // Outputs: 9
13. Object literal shorthand:
Shorter syntax for defining object properties and methods
const x = 2, y = 3;
const obj = {
x,
y,
toString() {
return `{x: ${x}, y: ${y}}`;
}
};
console.log(obj.toString()); // Outputs: {x: 2, y: 3}
14. Iterators and for...of loop:
New iterable objects and a way to loop over them
const iterable = ['a', 'b', 'c'];
for (const value of iterable) {
console.log(value);
} // Outputs: a, b, c
15. Generator functions:
Special type of function that can be stopped and resumed later
function* idGenerator() {
let id = 0;
while (true) {
yield ++id;
}
}
const gen = idGenerator();
console.log(gen.next().value); // Outputs: 1
console.log(gen.next().value); // Outputs: 2
16. Array and Object methods:
New methods added to work with arrays and objects
const array = [1, 2, 3, 4, 5];
console.log(array.includes(3)); // Outputs: true
const obj = {
a: 1,
b: 2,
c: 3
};
// Outputs: [['a', 1], ['b', 2], ['c', 3]]
console.log(Object.entries(obj));
17. Proxy:
A special object that "wraps" another object and can intercept actions performed on the wrapped object
const target = {};
const handler = {
get: (obj, prop) => prop in obj ? obj[prop] : 0
};
const proxy = new Proxy(target, handler);
console.log(proxy.nonExistingProperty); // Outputs: 0
These should give us a comprehensive overview of the changes and additions to ECMAScript in its latest versions.